I was writing a little function in Ruby and thought I’d ask ChatGPT to have a go at it.
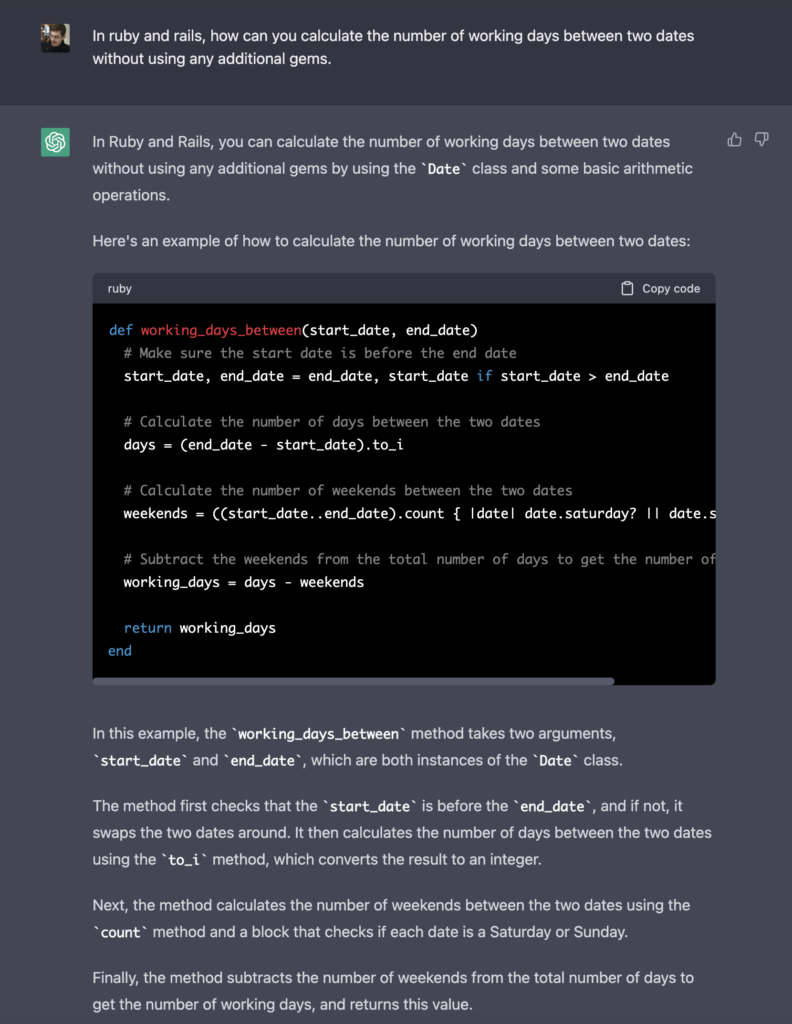
It is easy to read, explicit, and fairly idiomatic Ruby (though not concise). Not the most performant implementation but nothing terrible. It also mainly works but does have a bug in some cases.
Here is the code:
def working_days_between(start_date, end_date)
# Make sure the start date is before the end date
start_date, end_date = end_date, start_date if start_date > end_date
# Calculate the number of days between the two dates
days = (end_date - start_date).to_i
# Calculate the number of weekends between the two dates
weekends = ((start_date..end_date).count { |date| date.saturday? || date.sunday? })
# Subtract the weekends from the total number of days to get the number of working days
working_days = days - weekends
return working_days
end
If you have the start/end date on a weekend, then you get a negative answer. Eg,
working_days_between(Date.parse("Sat, 04 Mar 2023"), Date.parse("Sun, 05 March 2023"))
=> -1
It is because the weekend number of days calculation is including both the start date and the end date. Ie, working_days = 1 – 2 = -1
A human could easily have made the same mistake, mind you.
A better / simpler implementation is:
(from_date...to_date).count { |date| date.on_weekday? }
Note the 3 dots (…) for the date range, which does not include the end date.
Later, I tried asking ChatGPT to regenerate the answer multiple times. It gave me quite a different version every time – some versions with bugs, some with no functions, some with support for public holidays, etc.
Leave a Reply